The 0.96-inch OLED display is a versatile and beginner-friendly component. It allows you to display text, graphics, and animations on a small screen, perfect for various Arduino projects. This guide will introduce you to the basics of working and including how to wire it up. Also, install necessary libraries, and code your Arduino to display custom messages or designs.
To begin, you’ll need an OLED 0.96 display, an Arduino board, and a few jumper wires to make the connections. It displays uses I2C communication, so connecting it to your Arduino requires only four wires: VCC, GND, SDA (data), and SCL (clock). Once everything is wired, you’ll install the Adafruit SSD1306 and GFX libraries in the Arduino IDE, which provide essential functions for controlling the OLED display’s graphics and text.
Finally, you’ll dive into the code to bring your OLED display to life. This tutorial will walk you through displaying simple text, drawing shapes, and even creating small animations. By the end of this tutorial, you’ll have a solid foundation for using OLEDs in your projects. Be ready to explore more advanced applications, whether it’s for a clock, a sensor display, or just a fun message screen!
Components:
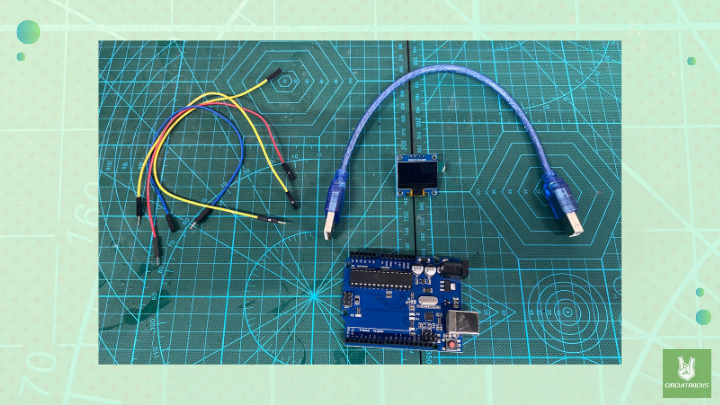
Connection:
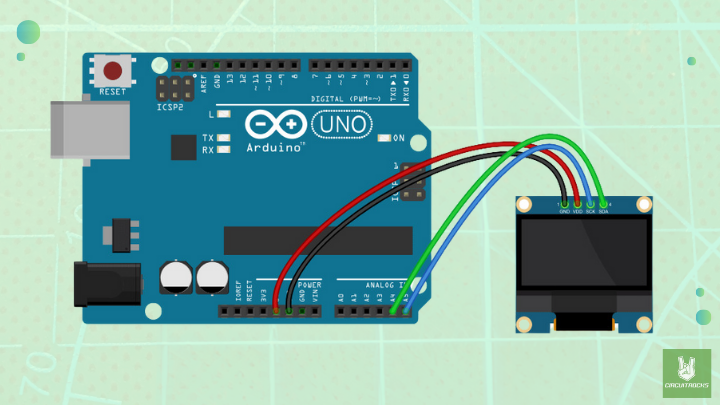
- VCC – Connects to the 5V pin on the Arduino to power the display.
- GND – Connects to the GND pin on the Arduino.
- SDA – Connects to A4 on an Uno.
- SCL – Connects to A5 on an Uno.
Notes:
You’ll need to install the following libraries:
- Adafruit SSD1306 – This library is specifically for OLED displays that use the SSD1306 driver chip.
- Adafruit GFX Library – This library provides core graphics functions (like drawing shapes and text) that the SSD1306 library relies on.
Code:
This code is designed to display a simple message, “I Love Circuitrocks,” on a 128×32 OLED screen connected to an Arduino.
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 32 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
#define SCREEN_ADDRESS 0x3C ///< See datasheet for Address; 0x3D for 128x64, 0x3C for 128x32
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
void setup() {
Serial.begin(9600);
// Initialize OLED display
if(!display.begin(SSD1306_SWITCHCAPVCC, SCREEN_ADDRESS)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;); // Stop if display initialization fails
}
display.clearDisplay();
// Set text size and color
display.setTextSize(1); // Smaller text size
display.setTextColor(SSD1306_WHITE);
display.setCursor(10, 10); // Adjust cursor position for center alignment
// Display message
display.println("I Love Circuitrocks");
// Show the buffer on the display
display.display();
}
void loop() {
// Nothing needed here as we're just displaying static text
}