The Arduino Melody Player with Speaker project becomes even more engaging with the use of speaker from Adafruit. The Mini Metal speaker quality ensures that your melody plays smoothly, making it an essential component of the project. This compact and reliable speaker is perfect for the project, providing clear and consistent audio output. By simply connecting the speaker’s wires to the Arduino, you can create a melody playback system. It demonstrates how even a basic setup can produce impressive sound results.
In this project, the Arduino generates specific frequencies that correspond to musical notes. Allowing you to bring your project to life with sound. The Arduino plays each note for a duration specified by another array, which determines the length of each note. A short delay between notes ensures that they are distinct and do not overlap, creating a clear and pleasant melody. The use of basic concepts such as arrays and loops. Make this project a learning tool for beginners who want to explore the basics of sound generation.
Understanding how to use this mini metal speaker with your Arduino. Opens up a wide range of possibilities for more advanced audio projects. The skills gained from this project can be applied to create even more complex projects. By mastering it, you lay the foundation for exploring the broader world of audio electronics. Making this project a crucial stepping stone for anyone interested in combining technology with music.
Components:
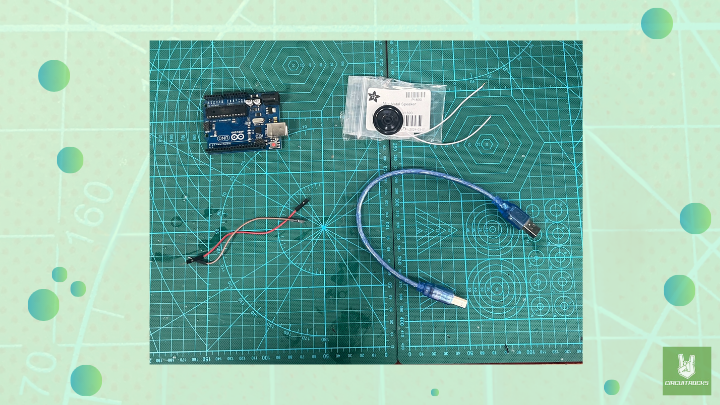
Connections:
- Speaker Positive (+) Wire to Arduino Pin 8: Connect the positive (usually red) wire of the M2850-8B-0L03R speaker to digital pin 8 on the Arduino. This pin will output the tone signals generated by the Arduino.
- Speaker Negative (-) Wire to Arduino Ground (GND): Connect the negative (usually black) wire of the speaker to one of the grounds (GND) pins on the Arduino. This completes the circuit and allows the speaker to function properly.
Additional Notes:
You might want to add a small resistor, if the sound is too quiet or distorted. Between the speaker’s positive wire and pin 8 to limit the current and protect the Arduino pin.
You can use a small amplifier circuit between the Arduino and the speaker for louder sound. For basic melody playback, direct connection should be sufficient.
Code:
#include "pitches.h"
// notes in the melody:
int melody[] = {
NOTE_C4, NOTE_G3, NOTE_G3, NOTE_A3, NOTE_G3, 0, NOTE_B3, NOTE_C4
};
// note durations: 4 = quarter note, 8 = eighth note, etc.:
int noteDurations[] = {
4, 8, 8, 4, 4, 4, 4, 4
};
void setup() {
// iterate over the notes of the melody:
for (int thisNote = 0; thisNote < 8; thisNote++) {
// to calculate the note duration, take one second divided by the note type.
//e.g. quarter note = 1000 / 4, eighth note = 1000/8, etc.
int noteDuration = 1000 / noteDurations[thisNote];
tone(8, melody[thisNote], noteDuration);
// to distinguish the notes, set a minimum time between them.
// the note's duration + 30% seems to work well:
int pauseBetweenNotes = noteDuration * 1.30;
delay(pauseBetweenNotes);
// stop the tone playing:
noTone(8);
}
}
void loop() {
// no need to repeat the melody.
}