Make your robots move using DC motors. In this article, we’ll take a look at DC motor fundamentals and how you control them using the L298N DC motor driver module.
DC Motors
DC motor (Direct Current) is the simplest type of motor. It has two leads: one positive and one negative. Regardless of polarity, the motor spins as soon as you connect these two leads on a power supply that matches its voltage requirement. The only difference is its rotation direction.
Controlling DC Motors
You can control a DC motor in these two aspects:
- Speed
- Spin Direction
With the combination of these two, you can alter your DC motor’s performance to fit your desired application.
PWM (Pulse Width Modulation)
PWM or Pulse Width Modulation is used to adjust the speed of a DC motor. It is a technique that allows us to regulate the average voltage input using ON and OFF pulses. These pulses are generally at a fast rate. Moreover, the average voltage depends on the duty cycle (time on overtime off).
The higher the duty cycle, the higher the average voltage input, the faster the DC motor is. Meanwhile, the lower the duty cycle, the lower the average voltage input, the slower the DC motor is.
Thankfully, Arduino has designated PWM pins. The PWM makes it easier to regulate DC motor speed. However, these pins only produce low power PWM signals. The amplifying transistor is required to operate appropriately. Connect the PWM pin to the base of a BJT or gate of a FET to drive the high power DC motor. There’s a more accessible alternative, though. More on that later.
H-bridges
On the other hand, H-bridges changes the spin direction of a DC motor. It is a circuit composed of four switches and a DC motor in the middle. Closing a pair of switches at the same time changes the direction of the current flow, changing the direction of rotation.
With these two techniques, you’ll gain complete control of a DC motor, where motor drivers enter. Motor drivers are circuit boards especially made for controlling DC motors. Within it are embedded H-bridge circuits and PWM control. The most common motor driver for Arduino is the L298N.
L298N Motor Driver IC
The L298 is a high voltage and current dual full-bridge driver designed to accept standard TTL logic levels and drive inductive loads such as relays, solenoids, DC and stepping motors. They are usually in 15- lead Multiwatt and PowerSO20 packages.
Dual full-bridge means it can support two DC motors at the same time. Pretty nifty, especially for 2WD robots.
Additionally, it has a peak current of 2A and an input voltage of 5 to 35V.
Pinout
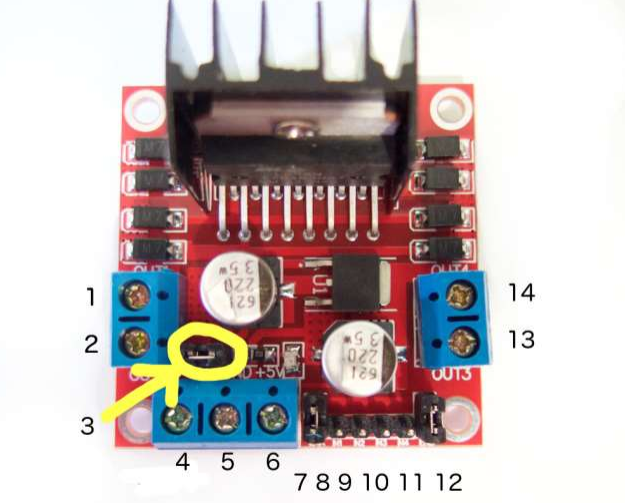
VCC (4) pin is where you connect the positive pin of your power supply. Input voltage can be between 5-35V.
GND (5) is a ground pin.
5V pin (6) is an output voltage pin for powering up your Arduino.
ENAand ENB(7 and 12) pins are to control the speed of Motor A and Motor B, respectively. Keeping the jumper in place (HIGH) makes the motor spin while disconnecting it (LOW) makes the motor stop. Connect to Arduino PWM output to control motor speed.
IN1 & IN2 (8 and 9) / IN3 & IN4(10 and 11) pins are to control the rotation direction of Motor A and Motor B, respectively. If both pins are both LOW or HIGH, the designated motor stops. On the other hand, if one pin is HIGH and the other is LOW, it either rotates clockwise or counterclockwise.
OUT A (1 and 2) are pins connected to Motor A.
OUT B (13 and 14) are pins connected to Motor B.
Programming
The Arduino program for controlling a DC motor using the L298N driver is very straightforward. You don’t even need a library.
// Motor A connections
int enA = 9;
int in1 = 8;
int in2 = 7;
// Motor B connections
int enB = 3;
int in3 = 5;
int in4 = 4;
void setup() {
// Set all the motor control pins to outputs
pinMode(enA, OUTPUT);
pinMode(enB, OUTPUT);
pinMode(in1, OUTPUT);
pinMode(in2, OUTPUT);
pinMode(in3, OUTPUT);
pinMode(in4, OUTPUT);
// Turn off motors - Initial state
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
digitalWrite(in3, LOW);
digitalWrite(in4, LOW);
}
void loop() {
directionControl();
delay(1000);
speedControl();
delay(1000);
}
// This function lets you control spinning direction of motors
void directionControl() {
// Set motors to maximum speed
// For PWM maximum possible values are 0 to 255
analogWrite(enA, 255);
analogWrite(enB, 255);
// Turn on motor A & B
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
digitalWrite(in3, HIGH);
digitalWrite(in4, LOW);
delay(2000);
// Now change motor directions
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
digitalWrite(in3, LOW);
digitalWrite(in4, HIGH);
delay(2000);
// Turn off motors
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
digitalWrite(in3, LOW);
digitalWrite(in4, LOW);
}
// This function lets you control speed of the motors
void speedControl() {
// Turn on motors
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
digitalWrite(in3, LOW);
digitalWrite(in4, HIGH);
// Accelerate from zero to maximum speed
for (int i = 0; i < 256; i++) {
analogWrite(enA, i);
analogWrite(enB, i);
delay(20);
}
// Decelerate from maximum speed to zero
for (int i = 255; i >= 0; --i) {
analogWrite(enA, i);
analogWrite(enB, i);
delay(20);
}
// Now turn off motors
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
digitalWrite(in3, LOW);
digitalWrite(in4, LOW);
}
Further, be sure to initialize ENA and ENB on PWM pins only. Also, this sketch only works for 2WD. If your hardware is a 4WD, you have to add more pins and adjust the program accordingly.
Finally, always make sure that your input voltage for the L298N is at least 2 volts greater than the minimum required for the motors. Some drivers become faulty when you feed it at a lower voltage.
That’s it for this time! Want to learn more? Check this article about ESP32 CAM with RTSP video streaming.