Overview
Ever wonder how it’s possible for smart watches to run for several weeks on a single charge?
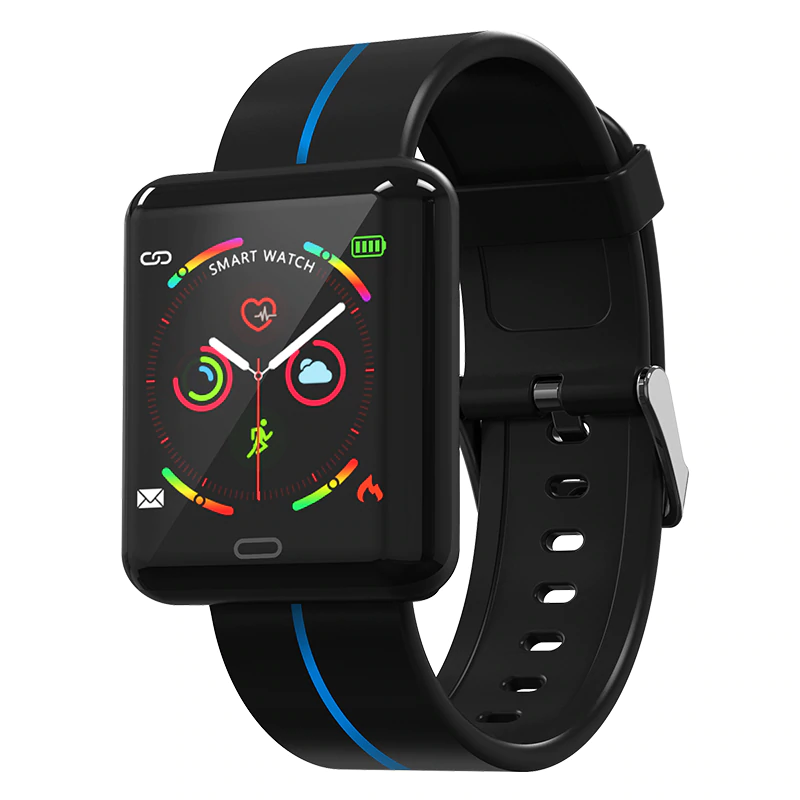
The answer is one of the most important features of microcontrollers – deep sleep.
Modern microcontrollers have different sleep modes which turn off CPU, memory and peripheral blocks in order to achieve progressively lower levels of power consumption.
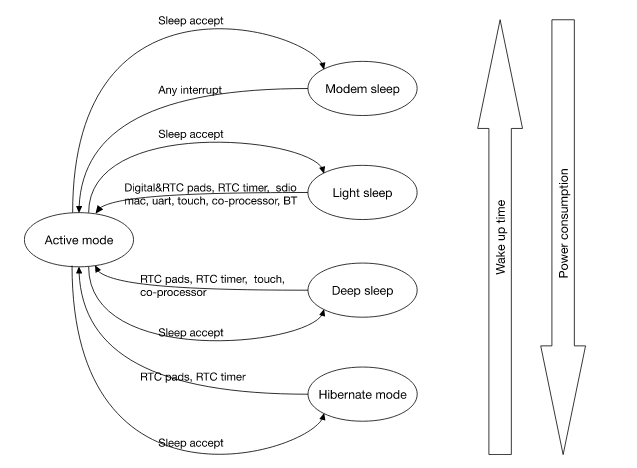
Going into an appropriate sleep mode is particularly important if you’re running off a battery. Doubly so if you’re using a WiFi enabled microcontroller. WiFi/BT subsystems use a lot of power.
Mode | ESP8266 | ESP32 |
Deep Sleep | 0.01mA | 0.01mA |
Sleep | 0.9mA | 0.8mA |
CPU | 15mA | 20mA |
WiFi RX | 56mA | 100mA |
WiFi TX | 320mA | 400mA |
These are power consumption figures gleaned from Espressif’s datasheets.
The increase in power consumption between the ESP32 running with the WiFi/BT block turned off (CPU only) and its deep sleep state is 2000x.
That’s the difference between running off a battery for over five straight years – and only having a single day of battery life.
This gap will only increase if you turn on the WiFi/BT block, as you would when transmitting or receiving data.
So if you’re planning to get any kind of meaningful battery life out of your device, you’ll have to learn how to get some (deep) sleep.
Components
Microcontroller
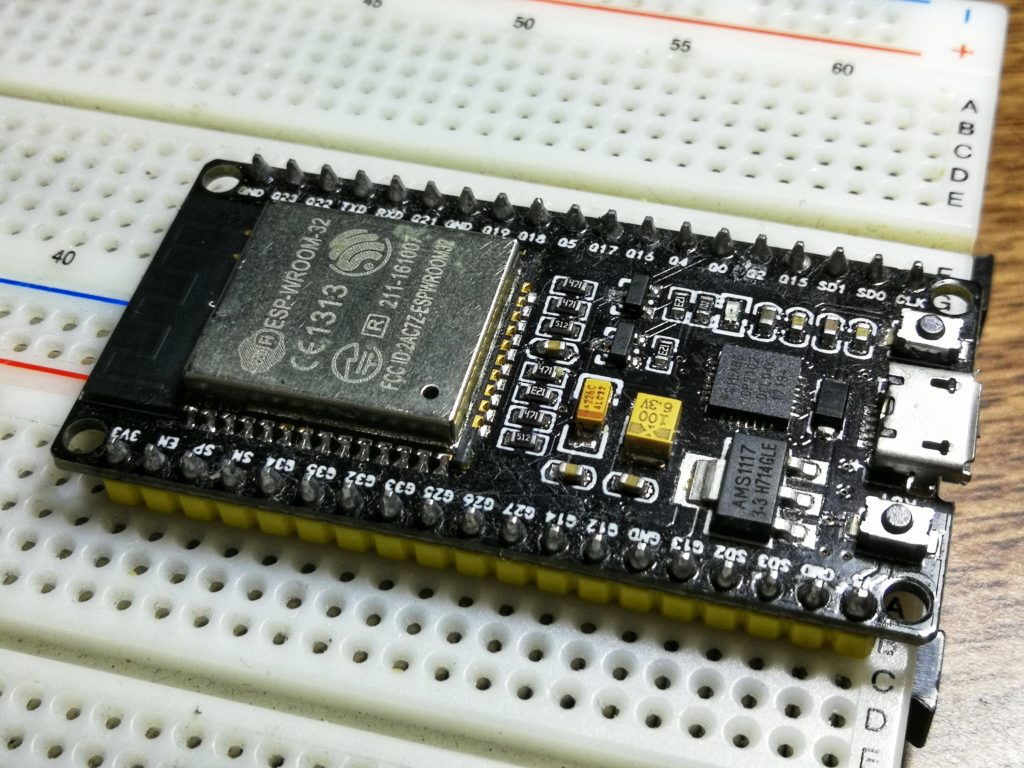
The ESP32 is a popular WiFi/BT enabled microcontroller with two Tensilisca Xtensa 32-bit LX6 cores running at 240MHz. The cores support dynamic frequency scaling, and you can configure the CPU to run at only 80MHz out of the box if you want to really save some power.
Software Setup
If you don’t have the ESP-IDF toolchain already installed, follow the instructions in this tutorial.
To configure the ESP32 core clock to run at 80MHz, run this command in your project directory to configure the ESP32 build.
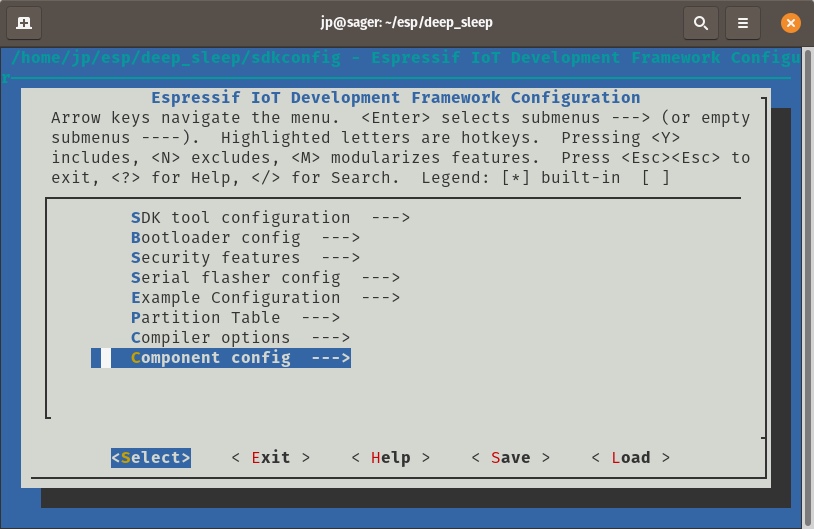
Go into the component config sub-menu. You should see a section which says “ESP32 specific”.
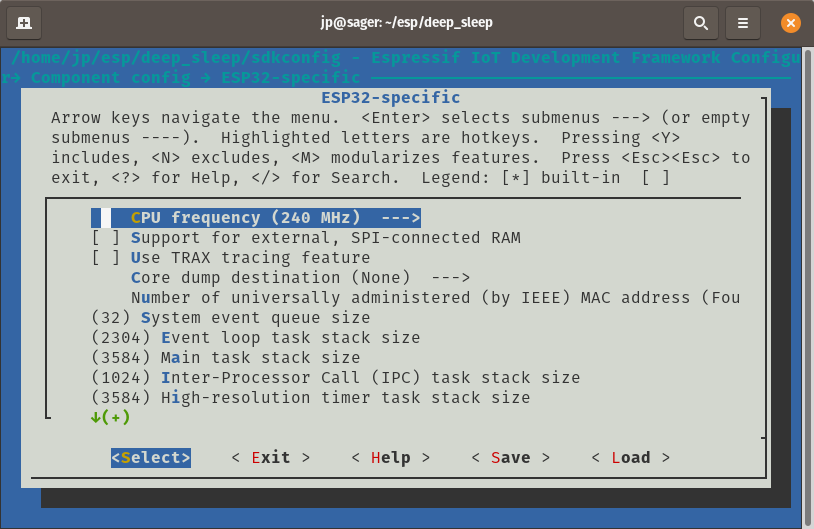
Now you can change the CPU frequency.
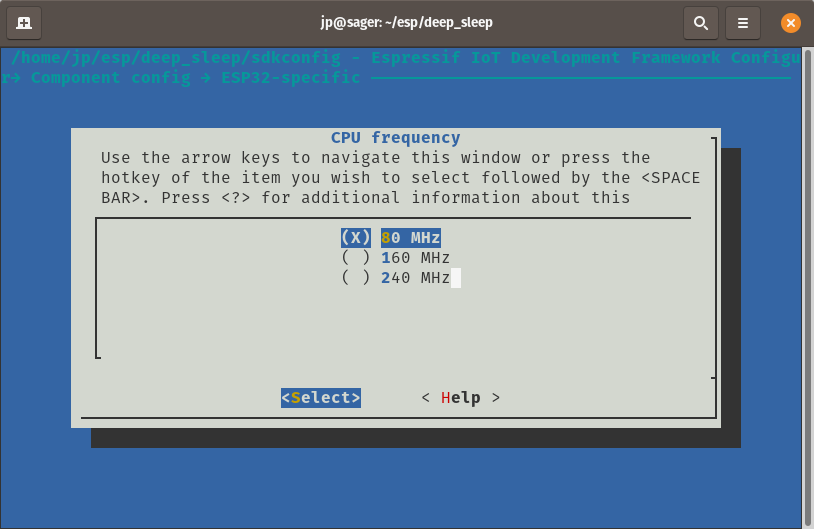
Setting it to 80MHz results in lower power consumption.
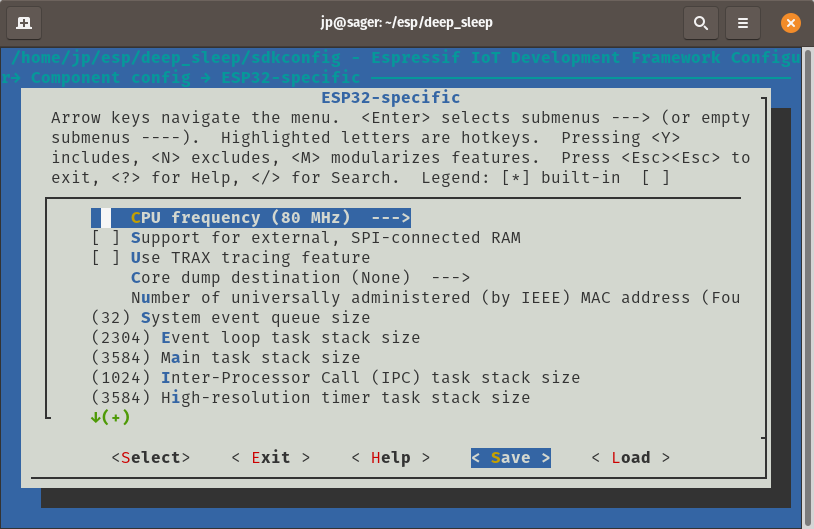
Now that you’ve set it to 80MHz, make sure to save your configuration settings.
Code
We’re going to make use of the ESP32’s built in capacitive touch sensor to wake up from deep sleep.
GPIO25 and GPIO26 will serve as capacitive touch enabled pins that will wake up the ESP32 if you touch them.
It’s a really cool feature to see in action.
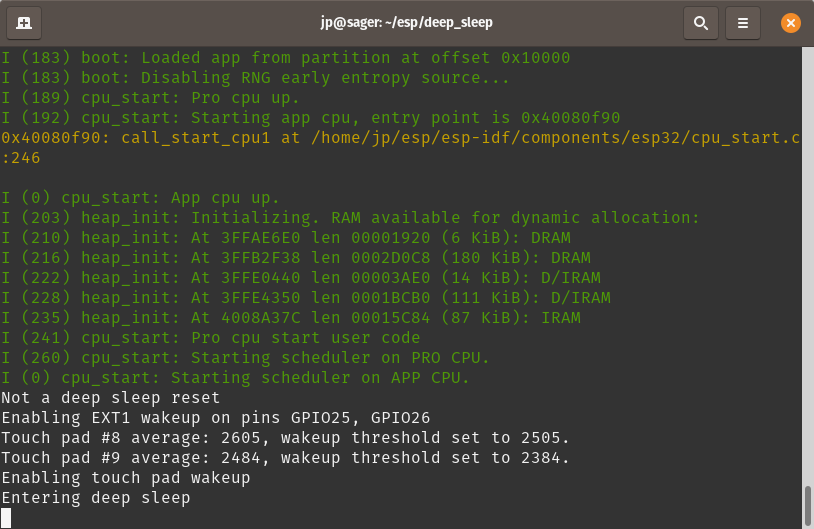
This should be the output of the serial monitor. “Not a deep sleep reset” indicates that it has yet to go into deep sleep. It should configure the touch pins and then immediately go to sleep.
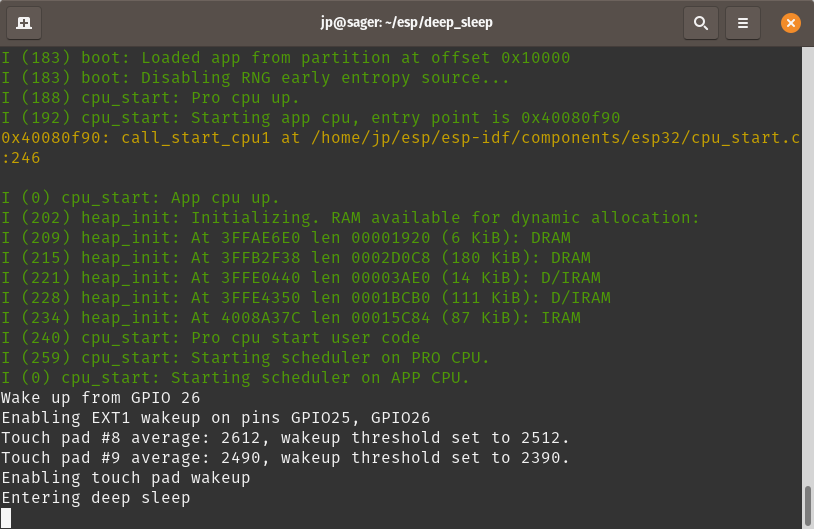
Touching either GPIO 25 or GPIO 26 should result in the ESP32 waking up momentarily, before going into deep sleep once again.

If you have an oscilloscope, you should be able to see the magnitude of difference in current consumption. Just use a low value resistor as a current shunt, then measure the voltage drop so you can see the current flowing into the ESP32.
Turning off logging or the serial debug feature of the ESP32 will also save a bit of additional power, but we’ll leave it on since this is how we’re getting output.
Using a dev board means there is some variability in power consumption figures. Some development boards will use a 3.3V regulator with truly atrocious quiescent current draw. This will heavily impact deep sleep figures. The ever popular AMS1117 used by most boards for example, will tend to draw several mA even without load. It is generally not suitable for a battery powered installation.
Some USB to Serial chips also don’t go into suspend mode or power off correctly when supplying power through the 5V pin.
These are just some of the possible culprits for seeing excessive current draw even in deep sleep mode.
That’s all folks!
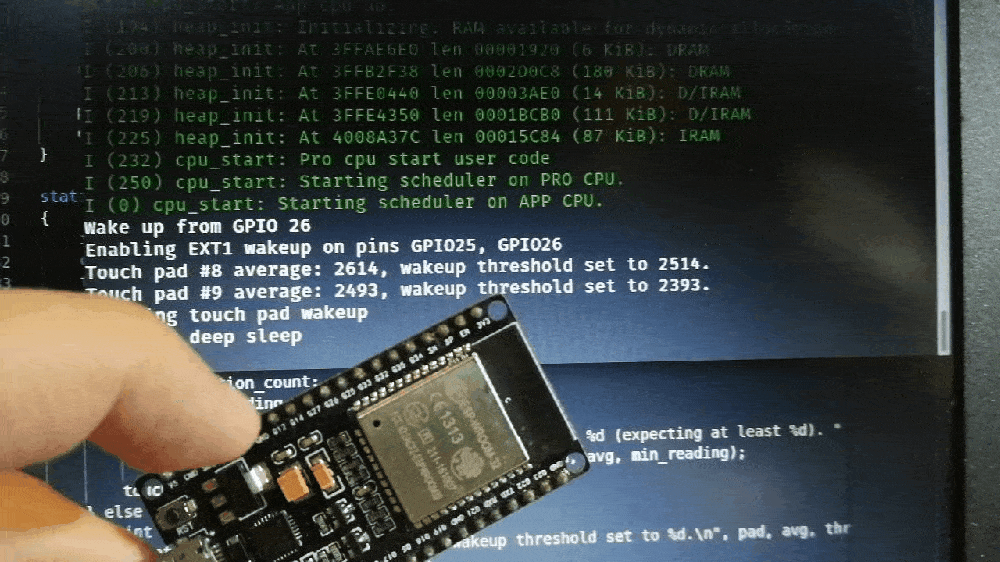
Congratulations! You’ve finally learned how to sleep (on a microcontroller).
Your battery operated projects should now last much longer.
Good luck, and stay creative!