Commencing on a journey to render dynamic visuals that will amaze people of all ages. This guide is meant for both beginners who want to dive into animated OLED displays operated by Arduino. Thanks to Arduino’s great platform and OLED screens which are clear and with high contrast. You can do animations you never thought about and never before even dreamed of. Whether you want to make your project more vibrant or you want to explore the capabilities of OLED technology. This guide facilitates you gaining the skills and knowledge to ultimately create your own lively animations. It open up opportunities for you to unleash your creativity alongside limitless potential of Arduino and OLED technology.
This project aims to explore the capabilities of OLED technology and Arduino’s to generate dynamic, eye-catching animations. It serves multiple purposes, from educational demonstrations of programming and electronics principles to decorative and entertainment applications. By cycling through different animations, this project will foster creativity but also provide a foundation for more complex projects. Whether for learning, decoration, or simply the joy of making. The dynamic OLED animation display promises to be a fun and rewarding into the world of digital art.
Components:
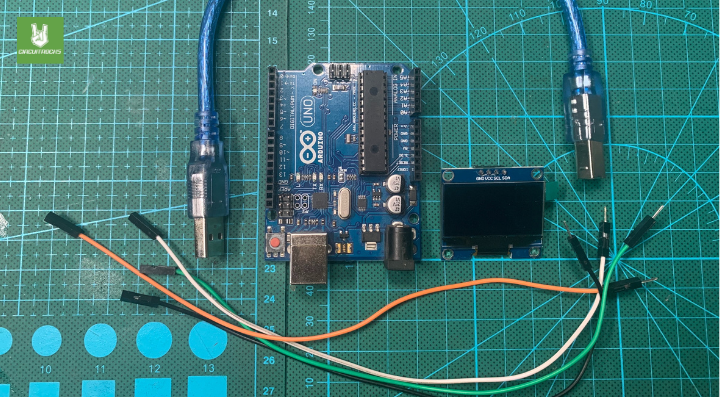
Connections:
OLED Display to Arduino via I2C:
- VCC of OLED to 5V on Arduino
- GND of OLED to GND on Arduino.
- SCL (Serial Clock) of OLED to A5 on Arduino Uno
- SDA (Serial Data) of OLED to A4 on Arduino Uno
Additional Notes:
- Ensure you have installed the necessary libraries (
Adafruit_GFX
andAdafruit_SH110X
or a library specific to the SH1106 if using that) in the Arduino IDE before uploading your code. - Your sketch involves drawing a cloud and moving raindrops. The
drawCloud
function and the logic in theloop
function handle these animations. - The raindrops are reset to the cloud’s position once they exceed the screen height, simulating continuous rain.
Code:
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SH110X.h>
#define i2c_Address 0x3C // OLED display I2C address, adjust if necessary
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin (not used with I2C interfaces)
Adafruit_SH1106G display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
#define NUM_DROPS 10
struct Raindrop {
int x, y;
int speed;
} drops[NUM_DROPS];
int cloudX = 0; // Initial position of the cloud
int cloudY = 10; // Fixed vertical position of the cloud
int cloudWidth = 40; // Width of the cloud
int cloudHeight = 20; // Height of the cloud
int cloudSpeed = 1; // Speed of cloud movement
bool movingRight = true; // Direction of cloud movement
void setup() {
Serial.begin(9600);
if (!display.begin(i2c_Address, true)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;); // Don't proceed, loop forever
}
display.display();
delay(2000); // Pause for 2 seconds
display.clearDisplay();
// Initialize raindrops with positions relative to the cloud and random speeds
for (int i = 0; i < NUM_DROPS; i++) {
drops[i].x = cloudX + random(cloudWidth);
drops[i].y = cloudY + cloudHeight;
drops[i].speed = random(1, 4); // Adjust for faster or slower drops
}
}
void drawCloud(int x, int y) {
// Simple cloud representation using filled circles
display.fillCircle(x + 10, y, 10, SH110X_WHITE);
display.fillCircle(x + 20, y - 5, 15, SH110X_WHITE);
display.fillCircle(x + 30, y, 10, SH110X_WHITE);
}
void loop() {
display.clearDisplay(); // Clear the display buffer
// Draw the cloud
drawCloud(cloudX, cloudY);
// Move the cloud
if (movingRight) {
cloudX += cloudSpeed;
if (cloudX > SCREEN_WIDTH - cloudWidth) movingRight = false; // Change direction
} else {
cloudX -= cloudSpeed;
if (cloudX < 0) movingRight = true; // Change direction
}
// Move and draw each raindrop
for (int i = 0; i < NUM_DROPS; i++) {
if (drops[i].y > SCREEN_HEIGHT) {
drops[i].y = cloudY + cloudHeight; // Reset to start from the cloud
drops[i].x = cloudX + random(cloudWidth) - cloudWidth / 2; // Recalculate X to follow the cloud
drops[i].speed = random(1, 4); // Reset speed
} else {
drops[i].y += drops[i].speed; // Continue falling
}
// Draw raindrop
display.drawPixel(drops[i].x, drops[i].y, SH110X_WHITE);
}
display.display(); // Show the display buffer on the screen
delay(50); // Update every 50 milliseconds for smoother animation
}
Troubleshooting:
- If the display doesn’t turn on or show the expected graphics, double-check the wiring, ensure the I2C address is correct, and verify that the display is powered correctly.
- If you encounter compilation errors related to the display initialization, confirm that you’re using the right class and library for your SH1106 OLED display. The code snippet mentions
Adafruit_SH1106G
, which might be a typo or mix-up with library classes.